Class java.awt.Component
- Direct Subclasses:
- Box.Filler, Button, Canvas, Checkbox, Choice, Container, Label, List, Scrollbar, TextComponent
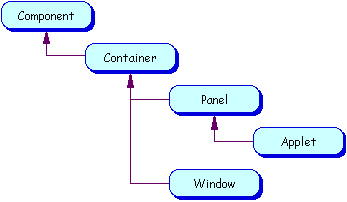
Every Container object has its own layout manager that know how to layout its Components.
For example, for the simplest container class Panals, the default layout manager is the FlowLayout layout manager.
//DefaultLayout.java
import java.awt.*;
import java.applet.Applet;
public class DefaultLayout extends Applet {
Button button1, button2, button3,
button4, button5, button6,
button7, button8, button9;
public void init() {
button1 = new Button("1");
button2 = new Button("2");
button3 = new Button("3");
button4 = new Button("4");
button5 = new Button("5");
button6 = new Button("6");
button7 = new Button("7");
button8 = new Button("8");
button9 = new Button("9");
add(button1);
add(button2);
add(button3);
add(button4);
add(button5);
add(button6);
add(button7);
add(button8);
add(button9);
}
}
GridLayout
//filename: Panels.java
import java.awt.*;
import java.applet.*;
public class Panels extends Applet
{
public Button button1, button2;
public Label label1, label2;
public TextField textField1;
public TextArea textArea1;
public Panel topLeftPanel, topRightPanel, topPanel, bottomPanel;
public void init()
{
button1 = new Button("button1");
button2 = new Button("button2");
label1 = new Label("label1");
label2 = new Label("label2");
textField1 = new TextField("textField1");
textArea1 = new TextArea("textArea1");
topLeftPanel = new Panel(new GridLayout(2, 1, 20, 20));
topRightPanel = new Panel(new GridLayout(2, 1, 20, 20));
topPanel = new Panel(new GridLayout(1, 2, 20, 20));
bottomPanel = new Panel(new GridLayout(2, 1, 20, 20));
setLayout(new GridLayout(2, 1, 20, 20));
topLeftPanel.add(textField1); topLeftPanel.add(button1);
topRightPanel.add(label1); topRightPanel.add(label2);
topPanel.add(topLeftPanel); topPanel.add(topRightPanel);
bottomPanel.add(textArea1); bottomPanel.add(button2);
add(topPanel); add(bottomPanel);
}
}
Mixing Layout Managers
//filename: MixedManagers.java
import java.awt.*;
import java.applet.*;
public class MixedManagers extends Applet
{
public Button ba, bb, bc, bd, b1, b2;
public Panel p3;
public void init( )
{
// Define the widgets
b1 = new Button ("1");
b1.setForeground(Color.yellow);
b1.setBackground(Color.blue);
b2 = new Button ("2");
b2.setBackground(Color.green);
b2.setForeground(Color.red);
ba = new Button("A");
bb = new Button("B");
bc = new Button("C");
bd = new Button("D");
p3 = new Panel();
// A grid layout for the applet
setLayout(new GridLayout(1, 3, 20, 20));
// A flow layout for the panel
p3.setLayout(new FlowLayout(FlowLayout.CENTER));
p3.add(ba); p3.add(bb); p3.add(bc); p3.add(bd);
add(b1); add(b2); add(p3);
}
}